Dev & Tech Notes
Terms & Conditions ©2005-2025 TJohns.co
Articles in this Category
Top Left Text cha
Web & App Development
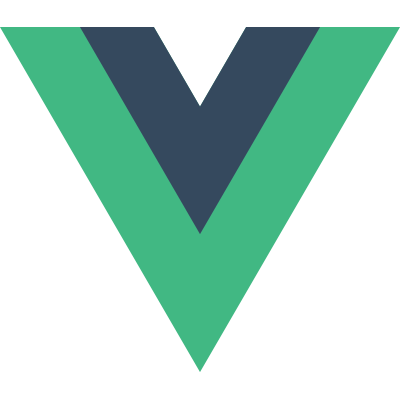
Vue(pronounced / vjuː /, like view) is a progressive framework for building user interfaces.Unlike other monolithic frameworks, Vue is designed from the ground up to be incrementally adoptable.The core library is focused on the view layer only, and is easy to pick up and integrate with other libraries or existing projects.On the other hand, Vue is also perfectly capable of powering sophisticated Single - Page Applications when used in combination with modern tooling and supporting libraries.
Visit VueJS.org- Details
- Written by Timothy Johns
- Category: Vue.js
- Hits: 994
Instance
Every Vue application starts by creating a new Vue instance...var vm = new Vue({
el: '#example',
data: '
var1: "hello",
var2: "world"
})
Template Stuff, Directives
insert into template:
<span>Message: {{ msg }}</span>
v-bind
<div v-bind:class="classObject"></div><div :class="classObj"></div>
div v-bind:style="{ color: activeColor, fontSize: fontSize + 'px' }"></div>
or cleaner:
<div v-bind:style="styleObject"></div>
data: { styleObject: { color: 'red', fontSize: '13px' } }
v-on
<div v-on:click="doSomething">
v-model
<div v-model
v-once
<span v-once>This will never change: {{ msg }}</span>
v-if
<h1 v-if="oneThing">Show One Thing</h1> <h1 v-else>Show Another Thing</h1>
on a template:
<template v-if="ok"> <h1>Title</h1> <p>Paragraph 1</p> <p>Paragraph 2</p> </template>
v-show
<h1 v-show="ok">Hello!</h1>
v-if is “real” conditional rendering because it ensures that event listeners and child components inside the conditional block are properly destroyed and re-created during toggles. v-if is also lazy: if the condition is false on initial render, it will not do anything - the conditional block won’t be rendered until the condition becomes true for the first time. In comparison, v-show is much simpler - the element is always rendered regardless of initial condition, with CSS-based toggling. Generally speaking, v-if has higher toggle costs while v-show has higher initial render costs. So prefer v-show if you need to toggle something very often, and prefer v-if if the condition is unlikely to change at runtime.
v-for
var app3 = new Vue ({
<div v-for="item of items"></div>